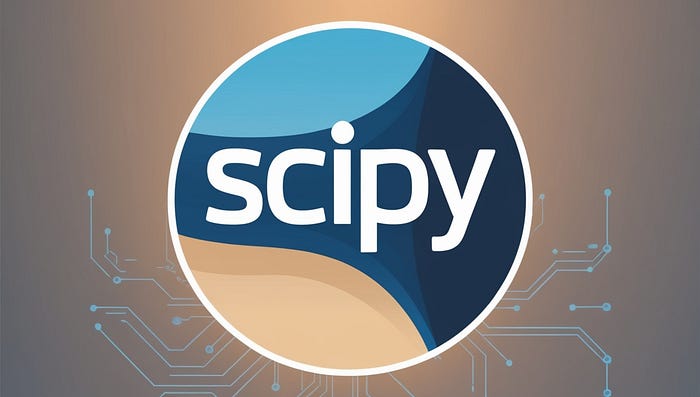
In an era where data reigns supreme, the ability to analyze and manipulate numerical information is more crucial than ever. Whether you’re a student delving into the world of scientific computing, a researcher seeking to streamline your data analysis, or a developer looking to enhance your projects with powerful libraries, understanding how to leverage tools like SciPy can be a game changer. SciPy, a cornerstone of the Python scientific ecosystem, is specifically designed for scientific and technical computing. It builds on the capabilities of NumPy and offers a vast array of functions for optimization, integration, interpolation, eigenvalue problems, algebraic equations, and much more.
In this blog post, we will guide you through the essential steps to get started with SciPy. We’ll cover the installation process, ensuring you have the library set up correctly on your system, and provide you with basic usage examples to illustrate its power and versatility. By the end of this post, you will be equipped with the foundational knowledge needed to begin exploring the rich functionalities that SciPy has to offer, empowering you to tackle your scientific computing challenges with confidence. Let’s dive in and unlock the potential of SciPy together!
Step-by-Step Instructions
SciPy is an open-source Python library used for scientific and technical computing. It builds on NumPy and provides a large number of mathematical algorithms and functions built on the NumPy extension. This guide will help you install SciPy, set it up, and use some of its basic features.
Step 1: Installing SciPy
Before you can use SciPy, you need to install it. The easiest way to install SciPy is using pip, Python’s package manager. Open your terminal or command prompt and type the following command:
pip install scipy
If you’re using Anaconda, you can install SciPy using conda:
conda install scipy
Step 2: Importing SciPy
Once you have installed SciPy, you can start using it in your Python scripts. To use SciPy, you need to import it. Here’s how you can do that:
import scipy
For specific functionalities, you may want to import submodules. For example, if you want to use the optimization functions, you can import the optimize module:
from scipy import optimize
Step 3: Basic Usage Examples
Example 1: Using scipy.optimize for Finding Roots
Let’s find the roots of a simple function, such as ( f(x) = x² — 4 ). The roots of this function are ( x = -2 ) and ( x = 2 ).
from scipy import optimize
# Define the function
def f(x):
return x**2–4
# Find the root
root = optimize.root(f, 0) # Starting guess is 0
print(f”Root: {root.x[0]}”)
Example 2: Using scipy.integrate for Numerical Integration
Suppose we want to compute the integral of ( f(x) = x² ) from 0 to 1.
from scipy import integrate
# Define the function
def f(x):
return x**2
# Compute the integral
integral, error = integrate.quad(f, 0, 1)
print(f”Integral of x² from 0 to 1: {integral}”)
Example 3: Using scipy.stats for Statistical Functions
You can also use SciPy for statistical analysis. For example, let’s compute the mean and standard deviation of a dataset.
from scipy import stats
data = [1, 2, 3, 4, 5]
mean = stats.tmean(data)
std_dev = stats.tstd(data)
print(f”Mean: {mean}, Standard Deviation: {std_dev}”)
So far we have covered the basics of getting started with SciPy. We went through the installation process, how to import the library, and looked at a few basic usage examples involving optimization, integration, and statistics.
Key Takeaways:
- SciPy is a powerful library for scientific computing in Python.
- Installation can be done easily using pip or conda.
- You can perform various tasks such as finding roots of functions, numerical integration, and statistical analysis with SciPy.
Now you’re ready to explore the vast capabilities of SciPy in your scientific computing projects!
Real-World Applications
When embarking on the journey of scientific computing, SciPy emerges as an invaluable tool. This powerful library for Python has become a cornerstone for researchers, engineers, and data scientists alike, enabling them to tackle complex problems with elegance and efficiency. The significance of SciPy extends far beyond mere calculations; it opens the door to a myriad of real-world applications that impact various industries and fields. Let’s delve into some of these applications, exploring how they shape our world and drive innovation.
1. Engineering and Simulation
In the realm of engineering, simulation is key. SciPy is widely used for solving differential equations that model physical systems, such as fluid dynamics or structural analysis. For instance, consider an aerospace engineer tasked with designing a new aircraft. They utilize SciPy to simulate airflow over the wings, allowing them to refine their design for optimal performance and safety. By employing functions from the scipy.integrate module, they can numerically solve the equations governing fluid motion, ultimately leading to advancements in aerodynamics.
2. Data Analysis in Finance
Finance is another sector where SciPy shines. Quantitative analysts, or “quants,” rely on it for tasks such as risk assessment and portfolio optimization. Imagine a financial analyst who needs to evaluate the potential returns of various investment strategies. Using SciPy’s optimization tools, they can identify the best asset allocation that maximizes returns while minimizing risk. A case study might involve a hedge fund employing SciPy to backtest trading algorithms, leading to more informed investment decisions and substantial profit margins.
3. Machine Learning and Artificial Intelligence
In recent years, the fields of machine learning and artificial intelligence have exploded in popularity, and SciPy plays a crucial role in this transformation. Researchers and developers use SciPy to preprocess data and perform numerical computations essential for training models. For example, when developing a neural network, one might utilize SciPy’s scipy.optimize to fine-tune hyperparameters, enhancing the model’s accuracy. A tech startup working on image recognition software may leverage SciPy alongside other libraries like NumPy and TensorFlow to streamline their development process, ultimately leading to innovative products that reshape industries.
4. Biomedical Research
The biomedical field also greatly benefits from SciPy’s capabilities. Scientists conducting research on disease spread or drug interactions utilize the library to analyze complex datasets. Consider a public health researcher studying the spread of infectious diseases. By employing SciPy to fit statistical models to epidemiological data, they can predict outbreak patterns and inform public health strategies. A notable case involved the use of SciPy to model the spread of COVID-19, helping policymakers allocate resources effectively during critical times.
5. Environmental Science
Lastly, environmental scientists use SciPy to model and analyze ecological data, aiding in conservation efforts and climate studies. For instance, a team studying climate change may use SciPy to process satellite data, analyzing temperature changes over time and their impact on ecosystems. By employing interpolation and optimization techniques from SciPy, they can create predictive models that guide conservation strategies and policy decisions.
From engineering and finance to machine learning and environmental science, SciPy serves as a vital asset across numerous industries. Its ability to facilitate complex calculations and data analysis empowers professionals to innovate and solve real-world problems efficiently. As you begin your journey with SciPy, consider the profound impact your work could have in these fields, driving progress and fostering solutions that benefit society as a whole.
Interactive Projects to Get Started with SciPy
Engaging in practical projects is one of the most effective ways to solidify your understanding of SciPy. By applying the concepts you’ve learned in a hands-on manner, you’ll gain confidence and deepen your knowledge of scientific computing in Python. Here are some interactive projects you can try on your own. Each project includes step-by-step instructions and expected outcomes to guide you through the process. Let’s dive in!
Project 1: Basic Statistical Analysis with SciPy
Objective: Analyze a dataset to compute basic statistics and visualize the results.
Step-by-Step Instructions:
- Install Required Libraries: Make sure you have SciPy and Matplotlib installed. You can do this via pip:
- pip install scipy matplotlib
- Import Libraries: Open your Python environment and import the necessary libraries:
- import numpy as np
import matplotlib.pyplot as plt
from scipy import stats
- Create or Load a Dataset: For simplicity, let’s create a random dataset:
- data = np.random.normal(loc=0, scale=1, size=1000) # 1000 samples from a normal distribution
- Compute Basic Statistics: Use SciPy to calculate mean, median, and standard deviation:
- mean = np.mean(data)
median = np.median(data)
std_dev = np.std(data) - print(f’Mean: {mean}, Median: {median}, Standard Deviation: {std_dev}’)
- Visualize the Data: Create a histogram to visualize the distribution:
- plt.hist(data, bins=30, density=True, alpha=0.6, color=’g’)
- # Plot a normal distribution curve
xmin, xmax = plt.xlim()
x = np.linspace(xmin, xmax, 100)
p = stats.norm.pdf(x, mean, std_dev)
plt.plot(x, p, ‘k’, linewidth=2)
plt.title(‘Histogram of Normally Distributed Data’)
plt.xlabel(‘Value’)
plt.ylabel(‘Frequency’)
plt.show()
Expected Outcome: You will see the mean, median, and standard deviation printed in your console, along with a histogram showing the distribution of your dataset superimposed with a normal distribution curve.
Project 2: Linear Regression with SciPy
Objective: Fit a simple linear regression model to a dataset and visualize the results.
Step-by-Step Instructions:
- Install Required Libraries: Ensure you have SciPy and Matplotlib:
- pip install scipy matplotlib
- Import Libraries: In your Python environment, import the necessary libraries:
- import numpy as np
import matplotlib.pyplot as plt
from scipy import stats
- Create Sample Data: Generate some sample data for the regression:
- np.random.seed(0)
x = np.random.rand(100) * 10 # Random x values
y = 2.5 * x + np.random.randn(100) # Linear relation with noise
- Perform Linear Regression: Use SciPy to perform linear regression:
- slope, intercept, r_value, p_value, std_err = stats.linregress(x, y)
- Make Predictions: Generate predictions from the regression model:
- y_pred = slope * x + intercept
- Visualize the Results: Plot the original data and the regression line:
- plt.scatter(x, y, label=’Data Points’)
plt.plot(x, y_pred, color=’red’, label=’Fitted Line’)
plt.title(‘Linear Regression Example’)
plt.xlabel(‘X’)
plt.ylabel(‘Y’)
plt.legend()
plt.show()
Expected Outcome: You will see a scatter plot of the data points along with a red line representing the fitted linear regression model, showcasing the relationship between the two variables.
Project 3: Signal Processing with SciPy
Objective: Apply a Fourier Transform to analyze the frequency components of a signal.
Step-by-Step Instructions:
- Install Required Libraries: Make sure you have SciPy and Matplotlib installed:
- pip install scipy matplotlib
- Import Libraries: In your Python environment, import the necessary libraries:
- import numpy as np
import matplotlib.pyplot as plt
from scipy.fft import fft
- Create a Sample Signal: Generate a signal composed of multiple frequencies:
- fs = 500 # Sampling frequency
t = np.linspace(0, 1, fs, endpoint=False) # Time variable
signal = 1.0 * np.sin(2.0 * np.pi * 50 * t) + 0.5 * np.sin(2.0 * np.pi * 120 * t) # Sum of two sine waves
- Compute the Fourier Transform: Apply the Fast Fourier Transform (FFT) to the signal:
- yf = fft(signal)
xf = np.fft.fftfreq(len(signal), 1 / fs)
- Visualize the Signal and Its Frequency Spectrum: Create plots for both the time domain and frequency domain:
- plt.subplot(2, 1, 1)
plt.plot(t, signal)
plt.title(‘Time Domain Signal’)
plt.xlabel(‘Time [s]’)
plt.ylabel(‘Amplitude’) - plt.subplot(2, 1, 2)
plt.plot(xf, np.abs(yf))
plt.title(‘Frequency Domain Signal’)
plt.xlabel(‘Frequency [Hz]’)
plt.ylabel(‘Magnitude’)
plt.xlim(0, 200) # Limit x-axis to 200 Hz
plt.tight_layout()
plt.show()
Expected Outcome: You will see the original signal plotted in the time domain, along with its frequency spectrum showing the magnitude of the different frequency components.
These interactive projects are designed to help you apply your knowledge of SciPy in practical situations. By completing these exercises, you’ll enhance your understanding of statistical analysis, linear regression, and signal processing. Don’t hesitate to experiment with the parameters and datasets to see how the results change. Happy coding!
Supplementary Resources
As you explore the topic of ‘Getting Started with SciPy’, it’s crucial to have access to quality resources that can enhance your understanding and skills. Below is a curated list of supplementary materials that will provide deeper insights and practical knowledge:
SciPy Installation Guide: https://scipy.org/install/
SciPy Tutorial: https://docs.scipy.org/doc/scipy/tutorial/index.html
Continuous learning is key to mastering any subject, and these resources are designed to support your journey. Dive into these materials to expand your horizons and apply new concepts to your work.
Elevate Your Python Skills Today!
Transform from a beginner to a professional in just 30 days with Python Mastery: From Beginner to Professional in 30 Days. Start your journey toward becoming a Python expert now. Get your copy on Amazon.
Explore More at Tom Austin’s Hub!
Dive into a world of insights, resources, and inspiration at Tom Austin’s Website. Whether you’re keen on deepening your tech knowledge, exploring creative projects, or discovering something new, our site has something for everyone. Visit us today and embark on your journey!