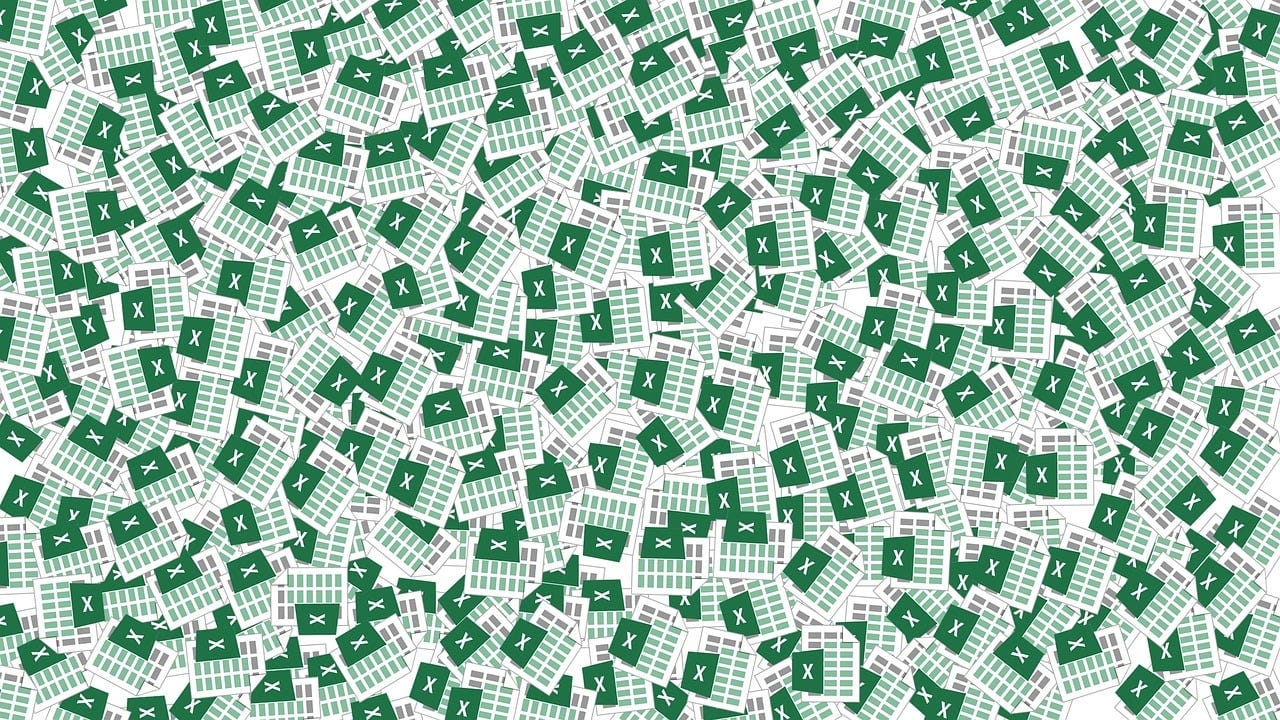
Excel VBA Tutorial: Your Comprehensive Guide to Mastering Automation in Excel
Welcome to our Excel VBA tutorial, where you’ll learn how to harness the power of Visual Basic for Applications (VBA) in Excel. Whether you’re a beginner looking to automate repetitive tasks or an advanced user seeking to optimize data management, this guide is designed for all skill levels. In this tutorial, we’ll cover the fundamentals of Excel VBA, provide practical examples, and help you unlock the full potential of this powerful tool.
What is Excel VBA?
Excel VBA is a programming language that enables users to automate tasks and create custom functions within Excel. It allows you to write macros, which are sets of instructions that can be run automatically to perform complex tasks efficiently. With VBA, you can:
- Automate repetitive tasks
- Create custom Excel functions
- Build interactive dashboards and forms
- Integrate Excel with other Microsoft Office applications
Getting Started with Excel VBA
Enabling the Developer Tab
Before diving into VBA programming, you’ll need to enable the Developer tab in Excel. Follow these steps:
- Open Excel and click on File.
- Select Options.
- In the Excel Options dialog, click on Customize Ribbon.
- Check the box next to Developer in the right pane.
- Click OK to save your changes.
Writing Your First Macro
Now that the Developer tab is enabled, let’s write your first macro:
- Go to the Developer tab and click on Visual Basic.
- In the VBA editor, click Insert and then Module to create a new module.
- In the code window, enter the following code:
Sub HelloWorld()
MsgBox "Hello, World!"
End Sub
This simple macro displays a message box with the text “Hello, World!” when executed.
- To run the macro, press F5 or click the Run button in the toolbar.
Understanding VBA Syntax
To effectively use Excel VBA, you need to understand its syntax. Here are some key concepts:
- Variables: Used to store data values. Declare a variable using
Dim
, like so:Dim myVar As String
. - Control Structures: Control the flow of your code using
If...Then
statements,For...Next
loops, and others. - Functions and Subroutines: Functions return values, while subroutines do not. Use
Function
for functions andSub
for subroutines.
Practical Examples of Excel VBA
Example 1: Automate Formatting
Let’s automate the formatting of a selected range of cells:
Sub FormatCells()
With Selection
.Font.Bold = True
.Interior.Color = RGB(255, 255, 0)
End With
End Sub
This macro changes the font to bold and sets the background color to yellow for the selected cells.
Example 2: Loop Through a Range
Here’s how to sum values in a range using a loop:
Sub SumRange()
Dim total As Double
Dim cell As Range
For Each cell In Selection
total = total + cell.Value
Next cell
MsgBox "Total: " & total
End Sub
This macro calculates the total of all selected cells and displays it in a message box.
Debugging Your VBA Code
Debugging is an essential skill when working with VBA. Here are some tips:
- Use breakpoints to pause code execution at a specific line.
- Utilize the Immediate Window to test expressions and output variable values.
- Don’t hesitate to use MsgBox statements to display variable values at different stages in your code.
More Resources for Excel VBA
If you’re eager to deepen your knowledge of Excel VBA, check out our comprehensive courses and training resources:
Conclusion
Excel VBA is a valuable tool that can significantly enhance your productivity and data management capabilities. By mastering VBA, you’ll be able to create powerful macros that automate complex tasks and simplify your workflow. Don’t forget to explore more advanced Excel features, such as those covered in our Macros Course, to truly unlock the potential of Excel.
To support our mission at Excel Foundations, consider donating or purchasing our ebook for further learning. Your contribution helps us provide valuable resources for Excel learners worldwide.
Next Steps
- Continue Learning VBA: Enroll in our comprehensive Excel VBA course to dive deeper into VBA programming and enhance your automation skills.
- Practice Coding: Set aside some time to practice writing your own macros. Try automating tasks you frequently perform in Excel to reinforce your learning.
- Explore Additional Resources: Check out our Top 5 Best Microsoft Excel Courses to further improve your Excel skills beyond VBA.
- Join the Community: Engage with other learners in Excel forums or social media groups to share your progress, ask questions, and learn from others’ experiences.
- Start a Project: Consider starting a personal project or a small business application that utilizes what you’ve learned in your VBA journey to solidify your knowledge.