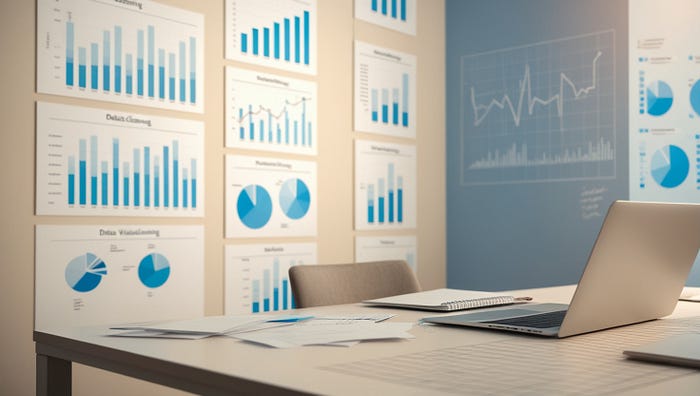
Pythonic Data Cleaning With NumPy
In the world of data science, clean data is the bedrock upon which robust analysis is built. Inaccurate or incomplete data can lead to flawed models and misguided conclusions. Python offers a variety of tools for data cleaning, with NumPy being one of the most efficient and powerful. This guide will take you through an introduction to data cleaning using NumPy, providing you with the skills needed to handle missing data, clean datasets, and perform fundamental data transformations.
Setting Up Your Environment: Installing NumPy
Before diving into data cleaning tasks, we need to set up our Python environment. The first step is to install NumPy. If you don’t have it already, you can easily install it using pip:
pip install numpy
With NumPy installed, you’re ready to start your journey into data cleaning.
Understanding Your Data: Initial Inspections
The first step in any data cleaning process is to understand the dataset you’re working with. Initial inspection involves loading the data and examining its structure:
import numpy as np
# Load a CSV file into a NumPy array
data = np.genfromtxt(‘data.csv’, delimiter=’,’, skip_header=1)
# View the first few rows of the dataset
print(data[:5])
By inspecting the first few rows, you can get a sense of the data types and the presence of any obvious issues such as missing values or anomalies.
Identifying Missing Data and Anomalies
Missing data and anomalies can wreak havoc on your analyses. Detecting them early helps in deciding on the best method to handle them:
# Identify missing data (represented by NaN in NumPy)
missing_data_mask = np.isnan(data)
num_missing = np.sum(missing_data_mask)
print(f”Number of missing entries: {num_missing}”)
Anomalies, like outliers, can often be identified using descriptive statistics:
mean = np.mean(data, axis=0)
std_dev = np.std(data, axis=0)
# Highlight potential anomalies
anomalies = np.any(abs(data — mean) > 3 * std_dev, axis=1)
print(f”Rows with anomalies: {np.where(anomalies)[0]}”)
Techniques for Handling Missing Values in NumPy
Handling missing values is crucial to maintaining data integrity. One approach is to remove rows or columns with missing data:
# Remove rows with missing data
cleaned_data = data[~np.isnan(data).any(axis=1)]
Another approach is to fill in missing values with a specific strategy, such as using the mean of the column:
mean_values = np.nanmean(data, axis=0)
filled_data = np.where(np.isnan(data), mean_values, data)
Replacing and Imputing Data Appropriately
Replacing invalid or irrelevant data with meaningful substitutes is another critical aspect of data cleaning. This can involve simple replacements or more sophisticated imputation techniques:
# Replace zeros with NaN (often zeros are placeholders for missing data)
data = np.where(data == 0, np.nan, data)
# Fill missing values with the median
median_values = np.nanmedian(data, axis=0)
data = np.where(np.isnan(data), median_values, data)
Standardizing Data Formats for Consistency
Data often comes in various formats that need to be standardized. For example, dates might be represented differently across rows or columns:
# Standardizing a dataset that includes dates
dates = np.array([‘2021–07–01’, ‘July 2, 2021’, ‘2021/07/03’], dtype=’str’)
# Convert all dates to a common format
standardized_dates = np.array([np.datetime64(date) for date in dates])
print(standardized_dates)
Removing Duplicates and Irrelevant Information
Duplicate entries can skew results and need to be removed. Similarly, irrelevant information should be filtered out. Identifying duplicates is straightforward with NumPy:
# Find unique rows and remove duplicates
unique_data = np.unique(data, axis=0)
Correcting Data Types and Formatting Issues
Data types should be consistent to avoid errors during analysis. NumPy helps in converting data types effortlessly:
# Convert all data to floating point numbers for consistency
data = data.astype(float)
Transforming Data for Analysis: Basic Operations
Once the data is clean, it often needs to be transformed for analysis. Basic transformations include scaling, normalizing, and aggregating:
# Normalize data to a 0–1 range
min_values = np.min(data, axis=0)
max_values = np.max(data, axis=0)
normalized_data = (data — min_values) / (max_values — min_values)
# Example of aggregating data
mean_values_per_column = np.mean(data, axis=0)
print(mean_values_per_column)
Advanced Data Cleaning Techniques with NumPy
More advanced techniques include handling categorical data, performing feature engineering, and using advanced imputation strategies. For instance, categorical data encoding can be done using NumPy:
# Encode categorical data
categories = np.array([‘A’, ‘B’, ‘C’, ‘A’, ‘B’])
unique_categories, encoded_data = np.unique(categories, return_inverse=True)
print(encoded_data)
Optimizing Performance: Efficient Data Cleaning
Efficiency is crucial when dealing with large datasets. NumPy’s vectorized operations are highly optimized for performance:
# Efficient computation using vectorized operations
squared_data = data ** 2
sum_squared = np.sum(squared_data, axis=0)
print(sum_squared)
Case Study: Real-world Example of Data Cleaning
Consider a dataset of customer transactions with missing values, outliers, and inconsistencies:
# Load dataset
data = np.genfromtxt(‘transactions.csv’, delimiter=’,’, skip_header=1)
# Identify and fill missing values
data = np.where(np.isnan(data), np.nanmean(data, axis=0), data)
# Normalize the relevant columns
normalized_data = (data — np.min(data, axis=0)) / (np.max(data, axis=0) — np.min(data, axis=0))
# Remove duplicates
cleaned_data = np.unique(normalized_data, axis=0)
Through these techniques, we transformed a messy dataset into one ready for meaningful analysis.
Best Practices and Common Pitfalls in Data Cleaning
Always back up your data before making changes and validate each step of your process. Common pitfalls include over-imputation of data and loss of important information while cleaning.
Conclusion: Mastering Data Cleaning with NumPy
Mastering data cleaning using NumPy empowers you to work efficiently with datasets, ensuring your analyses and models are built on a strong foundation. Whether you’re replacing missing values, standardizing formats, or transforming data, the techniques covered here provide a comprehensive toolkit for clean, reliable data analysis in Python.
Ready to elevate your Python skills? Transform from a beginner to a professional in just 30 days! Get your copy of ‘Python Mastery: From Beginner to Professional in 30 Days’ and start your journey to becoming a Python expert. Visit https://www.amazon.com/dp/B0DCL1F5J2 to get your copy today!
Explore more at Tom Austin’s Hub! Discover a wealth of insights, resources, and inspiration at Tom Austin’s Website. Whether you’re looking to deepen your understanding of technology, explore creative projects, or find something new and exciting, our site has something for everyone. Visit us today and start your journey!